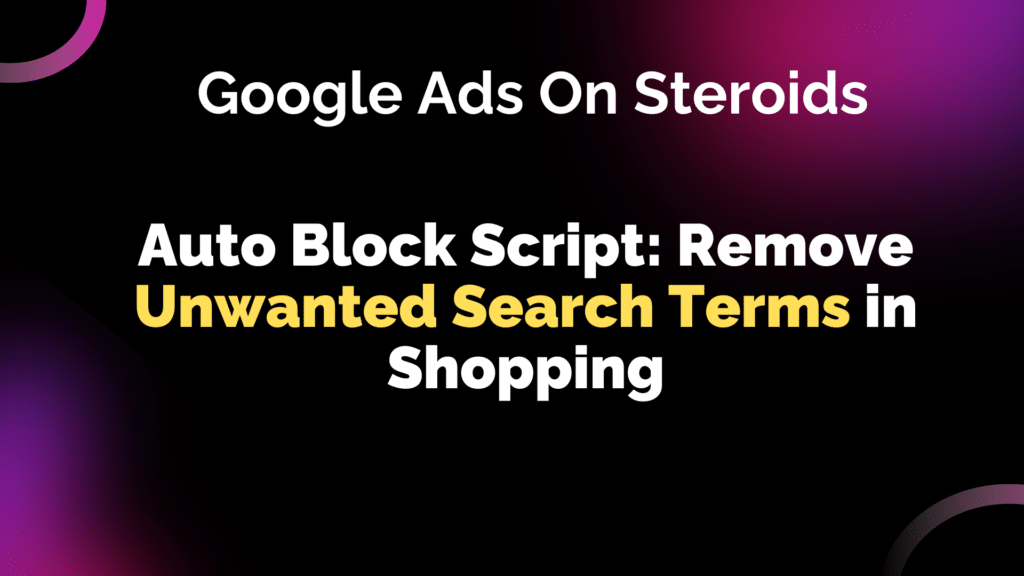
Imagine we’re selling remote controls for televisions. Naturally, we want the search terms to include the word “remote.” Without it, users might just be searching for a TV, not a remote control—especially if the search term report shows a lot of phrases containing only TV models.
This is exactly where the following Google Ads script comes in handy.
As PPC managers, our tasks are:
- Review shopping campaigns. We identify cases where missing a key word in search terms reduces their relevance for us and fails to drive conversions.
- Select the key word and configure it in the script. In this example, the keyword would be “remote.”
The script will then automatically add any search terms that don’t contain this word as exact match exclusions.
Configurations
1) Set up the REQUIRED_KEYWORDS list with the words you want to use. Each word should be in quotes and separated by a comma. You can add as many as you need.
2) Enter the name you want for the campaign label in CAMPAIGN_LABEL. This label will be used for the selected campaign(s).
3) Schedule the script to run once every morning.
Important: This script only changes shopping campaigns.
/*
Auto Block: Remove Unwanted Search Terms in Shopping
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
// --------------------------------------- Configuration:
const REQUIRED_KEYWORDS = ["YOUR KEYWORD HERE"];
const CAMPAIGN_LABEL = "YOUR LABEL HERE";
// --------------------------------------- End of the configuration
function main() {
ensureLabelExists();
const campaignIds = getEnabledShoppingCampaignIds();
const queriesToAddAsNegative = findNegativeKeywordCandidates(campaignIds);
if (queriesToAddAsNegative.length > 0) {
Logger.log(`Adding ${queriesToAddAsNegative.length} new negative keywords...`);
addNegativeKeywordsToCampaign(queriesToAddAsNegative);
} else {
Logger.log("No negative keyword additions required.");
}
}
function getEnabledShoppingCampaignIds() {
const campaignIds = [];
const campaignSelector = AdsApp.shoppingCampaigns()
.withCondition(`LabelNames CONTAINS_ANY ['${CAMPAIGN_LABEL}']`)
.withCondition("Status = ENABLED");
const campaigns = campaignSelector.get();
while (campaigns.hasNext()) {
const campaign = campaigns.next();
campaignIds.push(campaign.getId());
}
return campaignIds;
}
function findNegativeKeywordCandidates(campaignIds) {
const negativeCandidates = [];
const queryReport = AdsApp.report(
'SELECT Query FROM SEARCH_QUERY_PERFORMANCE_REPORT ' +
'WHERE CampaignId IN [' + campaignIds.join(",") + '] ' +
'DURING YESTERDAY');
const rows = queryReport.rows();
while (rows.hasNext()) {
const row = rows.next();
const query = row['Query'];
if (isNegativeKeywordCandidate(query)) {
negativeCandidates.push(query);
}
}
return negativeCandidates;
}
function isNegativeKeywordCandidate(query) {
return !REQUIRED_KEYWORDS.some(requiredWord => query.toLowerCase().includes(requiredWord));
}
function addNegativeKeywordsToCampaign(negativeQueries) {
const campaignIterator = AdsApp.shoppingCampaigns()
.withCondition(`LabelNames CONTAINS_ANY ['${CAMPAIGN_LABEL}']`)
.get();
if (campaignIterator.hasNext()) {
const campaign = campaignIterator.next();
Logger.log(`Selected campaign: ${campaign.getName()}`);
negativeQueries.forEach(query => {
campaign.createNegativeKeyword(`[${query}]`);
Logger.log(`${query} --> added as an exact negative keyword`);
});
}
}
function ensureLabelExists() {
const labelIterator = AdsApp.labels().withCondition(`Name = '${CAMPAIGN_LABEL}'`).get();
if (!labelIterator.totalNumEntities()) {
AdsApp.createLabel(CAMPAIGN_LABEL);
}
}
The script credit goes to: Krzysztof Bycina